I would like to welcome you to this blog and to thank you for taking the time to drop in. This will be my first blog post, so I thought I would start with something simple. In future posts I plan to build out from here and to extend the ideas into some more complex technologies and concepts such as Entity Framework, Flux patterns and of course how all of this can be rolled out on multiple platforms using .Net MAUI.
I don’t like to re-invent the wheel and I am a big fan of the Blazor components from Synfusion, so expect these components to feature quite often in these posts.
It is a fairly common requirement to want to make a C# Dropdown List from an enum in a single or multiselect dropdown. But what is the best way of achieving this, and how would this work in a multi-lingual application. I’m sure that the approach that I show here is not the only way of doing it, but I have found that this approach works well with both localization scenarios and where some code needs to be called when something is changed in the dropdown before any containing form is submitted.
Step 1
Install the Syncfusion Blazor extensions to Visual Studio.
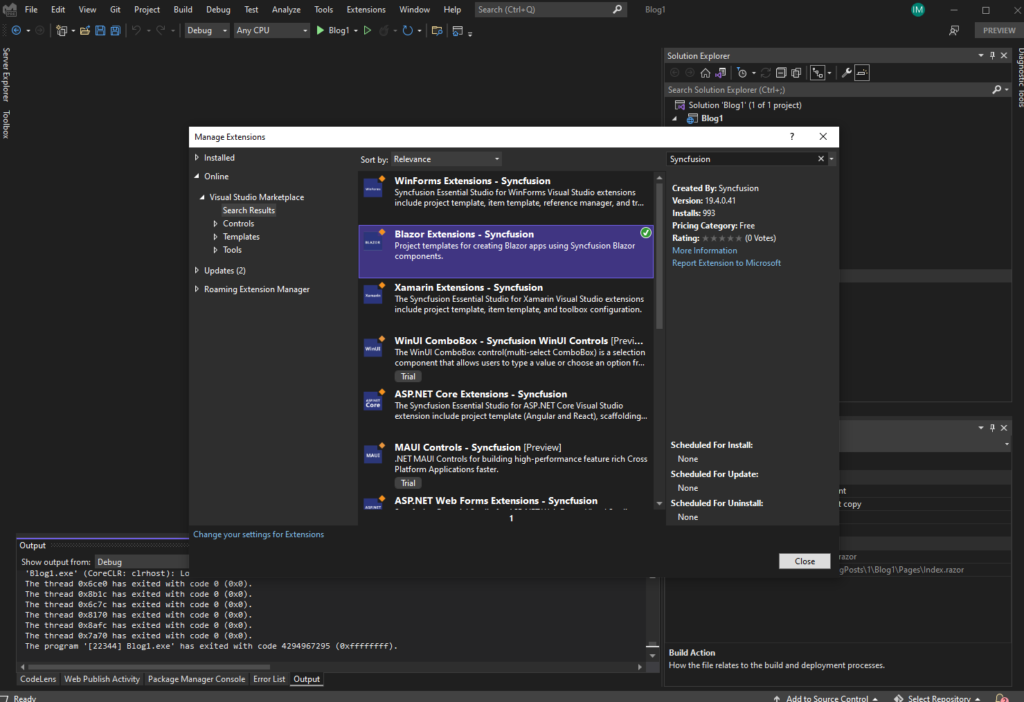
Step 2
Use the Syncfusion Extension to create a new Syncfusion Blazor Server App project (I used Bootstrap5 and .Net 6.0.)
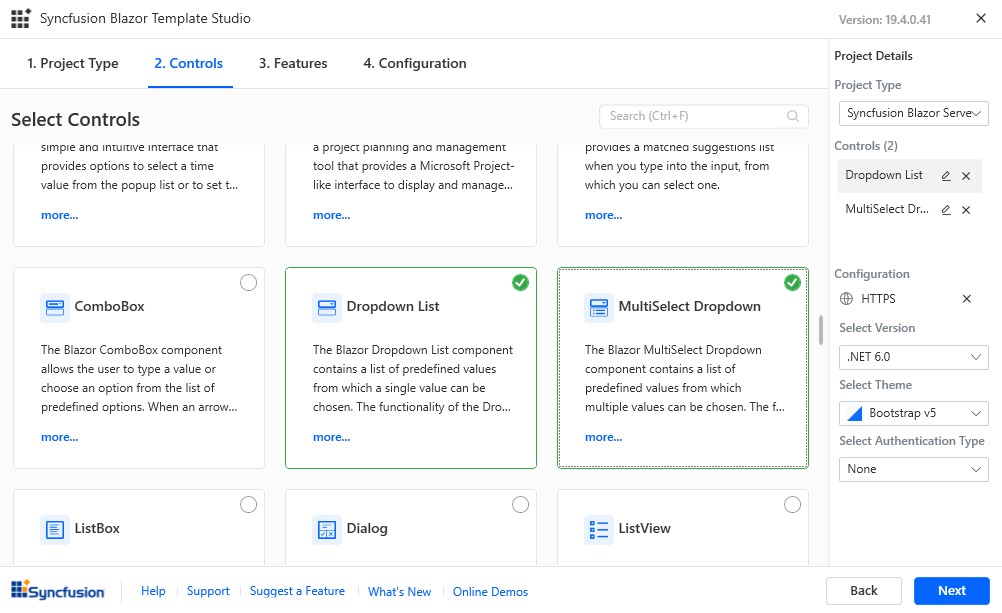
Step 4
Add a Resource (.resx) file to the Data folder and call it AppResources.resx.
Step 5
Add Name/Value pairs for the languages as shown below:
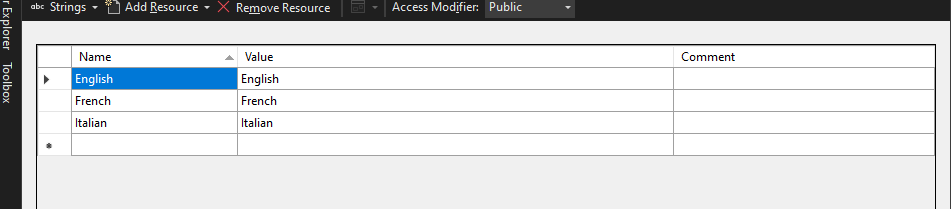
Step 6
Create a new Folder called Models and create a class called Language.cs containing the following code:
This code allows you to set other properties, such as Name or Locale/Culture codes etc. I think it is always good practice to put any text that will be seen by the user into a Resource (.resx) file. This means that it can be changed without searching through the code for it, but more importantly there are tools that I will discuss in later posts that allow a .resx file to be translated to multiple languages. I usually put the Resource File into its own project called Localization
using BlogPost1.Data;
namespace BlogPost1.Models;
public enum LanguageEnum { English, Italian, French, German }
public class Language
{
public Language(LanguageEnum languageEnum)
{
this.LanguageEnum = languageEnum;
}
public LanguageEnum LanguageEnum { get; set; }
public virtual string LanguageName
{
get
{
switch (LanguageEnum)
{
case LanguageEnum.English:
return AppResources.English;
case LanguageEnum.French:
return AppResources.French;
default:
return LanguageEnum.ToString();
}
}
}
}
Step 7
Replace the code in DropdownListFeatures.razor with the following
@page "/dropdownlist-features"
@using Syncfusion.Blazor.DropDowns
@using BlogPost1.Models
Properties
Selected Value
:@DropDownValue
Selected Text
:@ChangeValue
@code{
public List Languages = Enum.GetValues().Select(x => new Language(x)).ToList();
public LanguageEnum DropDownValue = LanguageEnum.English;
public LanguageEnum ChangeValue { get; set; } = LanguageEnum.English;
public void OnChange(Syncfusion.Blazor.DropDowns.ChangeEventArgs args)
{
this.ChangeValue = args.ItemData.LanguageEnum;
}
}
Step 8
Replace the code in MultiSelectDropdownFeatures.razor with the following
@page "/multiselectdropdown-features"
@using Syncfusion.Blazor
@using Syncfusion.Blazor.DropDowns
@using Syncfusion.Blazor.Data
@using BlogPost1.Models
MultiSelect Dropdown
Default Mode
Selected Languages:
@if(DropDownValues is not null)
{
- Values - @(string.Join
(",", DropDownValues.Select(x=>x.ToString() ?? "None").ToArray()))
}
@code{
public List Languages = Enum.GetValues().Select(x => new Language(x)).ToList();
public LanguageEnum[] DropDownValues = { };
public HashSet ChangeValues = new();
public void OnValueSelect(Syncfusion.Blazor.DropDowns.SelectEventArgs args)
{
ChangeValues.Add(args.ItemData.LanguageEnum);
DropDownValues = ChangeValues.ToArray();
}
public void ValueRemoved(Syncfusion.Blazor.DropDowns.RemoveEventArgs args)
{
ChangeValues.Remove(args.ItemData.LanguageEnum);
DropDownValues = ChangeValues.ToArray();
}
}
I hope that you find this post useful. I’d love to hear your comments. The code is available to download here.